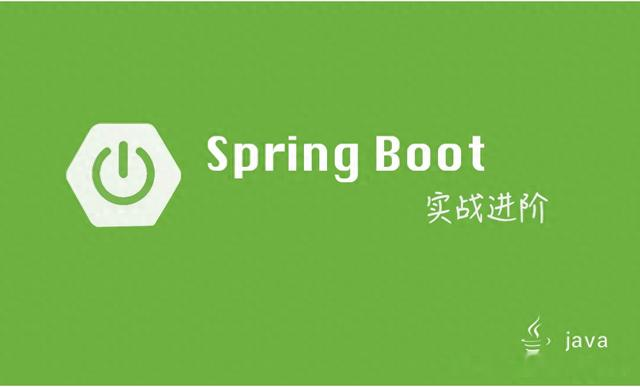
在 Spring Boot3 项目开发里,不少开发者会碰到 Cookie 值操作问题。按常规方法编写代码,获取或设置 Cookie 值时,却会出现报错、无法生效等情况,极大影响开发效率。那么,如何在 Spring Boot3 中正确对前端传递的 Cookie 值进行操作呢?
Spring Boot3 与 Cookie 操作的背景随着前后端分离架构的广泛应用,Spring Boot 凭借其出色的特性,成为后端开发的主流框架。Spring Boot3 更是在 Servlet 规范支持和依赖注入机制等方面进行了优化。而 Cookie 作为前后端数据交互中存储用户会话信息的常用工具,对其进行正确操作,关乎系统的安全与稳定。正因 Spring Boot3 的设计调整,开发者在处理 Cookie 值时容易陷入困惑。
获取 Cookie 值的方法与注意事项在 Spring Boot3 中,借助HttpServletRequest对象能获取请求中的 Cookie 信息。但需注意,request.getCookies()返回的数组可能为空,为避免空指针异常,要进行非空判断。示例代码如下:
import javax.servlet.http.Cookie;import javax.servlet.http.HttpServletRequest;@RestControllerpublic CookieController { @GetMapping("/getCookie") public String getCookie(HttpServletRequest request) { Cookie[] cookies = request.getCookies(); if (cookies != null) { for (Cookie cookie : cookies) { if ("cookieName".equals(cookie.getName())) { return cookie.getValue(); } } } return null; }}设置 Cookie 值及相关属性配置设置 Cookie 值,可借助HttpServletResponse对象在响应中添加 Cookie 信息。同时,还能设置 Cookie 的有效路径、过期时间等属性,精准控制 Cookie 的作用范围和生命周期。示例代码如下:
import javax.servlet.http.Cookie;import javax.servlet.http.HttpServletResponse;@RestControllerpublic CookieController { @GetMapping("/setCookie") public void setCookie(HttpServletResponse response) { Cookie cookie = new Cookie("cookieName", "cookieValue"); // 设置Cookie的有效路径为根路径 cookie.setPath("/"); // 设置Cookie在3600秒后过期 cookie.setMaxAge(3600); response.addCookie(cookie); }}保障 Cookie 安全性的加密操作为保障 Cookie 的安全性,可引入spring - security依赖,借助其加密功能对 Cookie 值进行加解密。
引入依赖
在pom.xml文件中添加spring - security相关依赖:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId></dependency>配置加密和解密密钥
为保证密钥的安全性,可将密钥配置在application.properties文件中,如cookie.encryption.key=yourSecretKey。在实际生产环境中,建议使用安全的密钥管理机制。
实现加解密接口
通过继承AbstractCookieEncoder和AbstractCookieDecoder抽象类,并重写相应方法实现 Cookie 的加解密。以下是简单的示例代码:
import org.springframework.security.web.util.UrlBase64Encoder;import org.springframework.security.web.util.UrlBase64Decoder;import org.springframework.web.util.CookieEncoder;import org.springframework.web.util.CookieDecoder;import java.nio.charset.StandardCharsets;public CustomCookieEncoder extends org.springframework.security.web.util.AbstractCookieEncoder { private final byte[] key; public CustomCookieEncoder(String key) { super(new UrlBase64Encoder()); this.key = key.getBytes(StandardCharsets.UTF_8); } @Override protected byte[] encodeValue(String value) { // 此处可添加自定义加密逻辑,例如AES加密 return value.getBytes(StandardCharsets.UTF_8); }}public CustomCookieDecoder extends org.springframework.security.web.util.AbstractCookieDecoder { private final byte[] key; public CustomCookieDecoder(String key) { super(new UrlBase64Decoder()); this.key = key.getBytes(StandardCharsets.UTF_8); } @Override protected String decodeValue(byte[] value) { // 此处可添加自定义解密逻辑,与加密逻辑对应 return new String(value, StandardCharsets.UTF_8); }}配置 Spring Security
在SecurityConfig类中,将自定义的CookieEncoder和CookieDecoder配置到 Spring Security 中:
import org.springframework.context.annotation.Bean;import org.springframework.context.annotation.Configuration;import org.springframework.security.config.annotation.web.builders.HttpSecurity;import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;import org.springframework.web.util.CookieEncoder;import org.springframework.web.util.CookieDecoder;@Configuration@EnableWebSecuritypublic SecurityConfig extends WebSecurityConfigurerAdapter { @Bean public CookieEncoder cookieEncoder() { return new CustomCookieEncoder("yourSecretKey"); } @Bean public CookieDecoder cookieDecoder() { return new CustomCookieDecoder("yourSecretKey"); } @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .anyRequest().permitAll() .and() .rememberMe() .cookieEncoder(cookieEncoder()) .cookieDecoder(cookieDecoder()); }}对 Cookie 进行全局配置的方法在 Spring Boot3 中,可利用ServletWebServerFactoryCustomizer接口对 Cookie 进行全局配置。该接口的原理是,在 Spring Boot 应用启动时,会自动扫描并执行实现了此接口的自定义配置类,从而对 Servlet 容器进行统一配置,其中就包括 Cookie 的相关设置。
以下是具体的代码示例:
import org.springframework.boot.web.servlet.server.ConfigurableServletWebServerFactory;import org.springframework.boot.web.servlet.server.ServletWebServerFactoryCustomizer;import org.springframework.stereotype.Component;@Componentpublic CookieCustomizer implements ServletWebServerFactoryCustomizer { @Override public void customize(ConfigurableServletWebServerFactory factory) { factory.addContextInitializers(initializer -> { initializer.addServletContextListener(servletContextEvent -> { // 设置默认Cookie的最大存活时间为2小时 int maxAge = 2 * 60 * 60; servletContextEvent.getServletContext().setInitParameter("org.apache.tomcat.util.http.ServerCookie.DEF_MAX_AGE", Integer.toString(maxAge)); // 设置默认Cookie的路径为根路径 servletContextEvent.getServletContext().setInitParameter("org.apache.tomcat.util.http.ServerCookie.DEF_PATH", "/"); }); }); }}在实际应用场景中,当多个服务模块都需要遵循统一的 Cookie 配置标准时,这种全局配置方式优势明显。例如在大型电商项目中,多个业务子系统之间需要共享用户的登录态 Cookie,通过全局配置,可以保证所有子系统生成的 Cookie 在路径、过期时间等关键属性上保持一致,避免因配置不一致导致的用户登录状态异常或数据共享问题。