示例
from abc import ABCMeta, abstractmethod# -------------------------------------------# 目标接口# -------------------------------------------class Payment(metaclass=ABCMeta): @abstractmethod def pay(self, money): passclass Alipay(Payment): def __init__(self, use_huabei=False): self.use_huabei = use_huabei def pay(self, money): if self.use_huabei: print(f'花呗支付{money}') else: print(f"支付宝支持{money}")class WechatPay(Payment): def pay(self, money): print(f"微信支付{money}")# -------------------------------------------# 要适配的类# -------------------------------------------class BankPay: def cost(self, money): print(f'银行卡支付{money}')class BitcoinPay: def cost(self, money): print(f'比特币支付{money}')# -------------------------------------------# 类适配器:# 缺点:# 每个类都需要有一个专门的适配器来适配,不适用于多个类适配# -------------------------------------------class NewBankPay(Payment, BankPay): def pay(self, money): self.cost(money)class NewBitCoinPay(Payment, BitcoinPay): def pay(self, money): self.cost(money)# -------------------------------------------# 对象适配器:适用于要适配的类很多的场景# -------------------------------------------class AdapterPayment(Payment): def __init__(self, payment): self.payment = payment def pay(self, money): self.payment.cost(money)# -------------------------------------------# client: 类适配器使用# -------------------------------------------print('=============类适配器=============')np = NewBankPay()np.pay(100)nbp = NewBitCoinPay()nbp.pay(200)# -------------------------------------------# client: 对象适配器使用# -------------------------------------------print('=============对象适配器=============')ap_bank = AdapterPayment(BankPay())ap_bank.pay(100)ap_bit = AdapterPayment(BitcoinPay())ap_bit.pay(200)运行结果
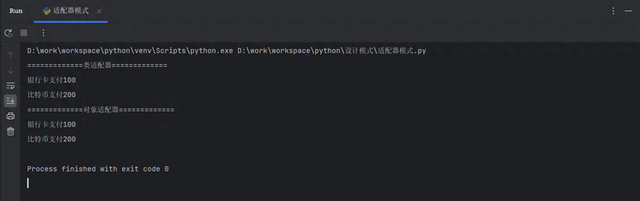