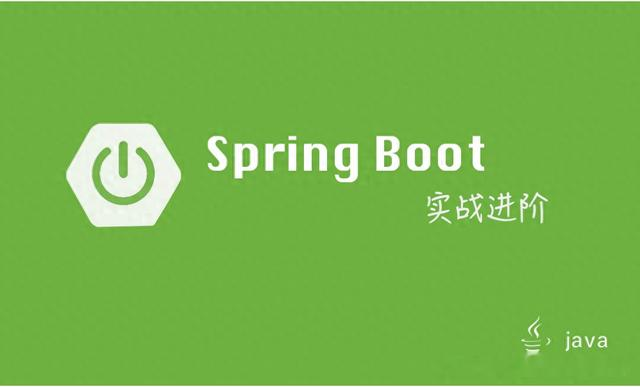
在使用 Spring Boot3 搭建项目时,不少开发者在借助 WebFlux 实现 RESTFul 接口的过程中遭遇阻碍。配置过程难题频发,接口响应速度也难以达到预期,极大地影响了开发效率。
Spring Boot3 与 WebFlux 应用现状Spring Boot 作为 Java 开发领域广泛应用的框架,持续迭代更新,Spring Boot3 带来的不仅是性能的显著提升,在功能层面同样实现了优化。WebFlux 作为 Spring 响应式编程的核心模块,能构建出高性能、低延迟的异步 Web 应用。而 RESTFul 凭借简洁性和可扩展性,成为接口设计的主流架构风格。尽管三者结合能打造出强大的 Web 服务,但在实际开发场景中,各类技术细节和兼容性问题依旧给开发者带来诸多困扰。
如何在 Spring Boot3 中用 WebFlux 实现 RESTFul 接口项目搭建
在着手实现接口前,首先要搭建一个 Spring Boot3 项目。通过 Spring Initializr 这一便捷工具,在其官网页面选择 Spring Boot3.0 版本,并勾选 WebFlux 依赖,便可一键生成项目基础骨架。创建完成后,在pom.xml文件中,能够看到 WebFlux 相关依赖已经自动添加:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-webflux</artifactId></dependency>编写控制器
新建一个控制器类,借助@RestController和@RequestMapping注解,能够精准定义 RESTFul 接口的路径和请求方法。以获取用户信息为例:
import org.springframework.http.MediaType;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RestController;import reactor.core.publisher.Mono;@RestController@RequestMapping("/users")public UserController { @GetMapping(produces = MediaType.APPLICATION_JSON_VALUE) public Mono<String> getUsers() { return Mono.just("{\"message\":\"获取用户信息成功\"}"); }}在上述代码中,@RestController表明该类为 REST 风格的控制器,@RequestMapping("/users")指定了接口的根路径。@GetMapping注解用于处理 HTTP GET 请求,produces = MediaType.APPLICATION_JSON_VALUE则指定了返回数据的格式为 JSON。
配置路由
使用RouterFunction配置路由,从而将请求映射到对应的控制器方法。在配置类中,编写如下代码:
import org.springframework.context.annotation.Bean;import org.springframework.context.annotation.Configuration;import org.springframework.http.MediaType;import org.springframework.web.reactive.function.server.RequestPredicates;import org.springframework.web.reactive.function.server.RouterFunction;import org.springframework.web.reactive.function.server.RouterFunctions;import org.springframework.web.reactive.function.server.ServerResponse;@Configurationpublic WebFluxConfig { @Bean public RouterFunction<ServerResponse> userRouterFunction(UserController userController) { return RouterFunctions.route( RequestPredicates.GET("/users").and(RequestPredicates.accept(MediaType.APPLICATION_JSON)), request -> ServerResponse.ok().bodyValue(userController.getUsers()) ); }}在这段配置代码里,RouterFunctions.route方法接收两个参数,第一个参数RequestPredicates.GET("/users").and(RequestPredicates.accept(MediaType.APPLICATION_JSON))定义了请求的路径和接受的数据格式,第二个参数指定了请求处理逻辑。
异常处理
在实际开发中,不可避免会遇到各种异常情况。为了提升应用的稳定性和用户体验,需要对异常进行统一处理。可以创建一个全局异常处理器,通过@ControllerAdvice和@ExceptionHandler注解实现:
import org.springframework.http.HttpStatus;import org.springframework.http.ResponseEntity;import org.springframework.web.bind.annotation.ControllerAdvice;import org.springframework.web.bind.annotation.ExceptionHandler;@ControllerAdvicepublic GlobalExceptionHandler { @ExceptionHandler(Exception.class) public ResponseEntity<String> handleException(Exception e) { return new ResponseEntity<>(e.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR); }}总结通过上述步骤,开发者能够较为系统地在 Spring Boot3 项目中使用 WebFlux 实现 RESTFul 接口。但在实际操作中,配置的微调、性能的优化等方面依然可能出现问题。如果你在开发过程中碰到任何难题,欢迎在评论区留言分享,大家一起探讨解决方案,共同提升开发能力。