在C#中,我们可以通过调用Windows API来找到其他应用程序的窗口句柄,以便进行与其交互的操作。下面我们将介绍如何使用C#来实现这一功能,并给出完整的示例代码。
查找窗口句柄我们可以使用Windows API中的FindWindow函数来查找指定类名和窗口名的窗口,并返回其句柄。下面是一个简单的示例代码:
FindWindow是一个用于查找指定类名和窗口名的窗口句柄的Windows API函数。下面是关于FindWindow函数的参数说明:
lpClassName:要查找的窗口类名。可以为null,表示不限定类名。lpWindowName:要查找的窗口标题(窗口名)。可以为null,表示不限定窗口名。函数原型如下:
[DllImport("user32.dll", SetLastError = true)]static extern IntPtr FindWindow(string lpClassName, string lpWindowName);lpClassName参数用于指定要查找的窗口类名。如果为null,则表示不限定类名,可以匹配任何类名的窗口。lpWindowName参数用于指定要查找的窗口标题(窗口名)。如果为null,则表示不限定窗口名,可以匹配任何窗口标题的窗口。FindWindow函数会返回找到的窗口句柄。如果未找到匹配的窗口,则返回IntPtr.Zero。
using System;using System.Runtime.InteropServices;class Program{ [DllImport("user32.dll", SetLastError = true)] static extern IntPtr FindWindow(string lpClassName, string lpWindowName); static void Main() { stringName = "Notepad"; // 要查找的窗口类名 string windowName = "Untitled - Notepad"; // 要查找的窗口标题 IntPtr hWnd = FindWindow(className, windowName); if (hWnd == IntPtr.Zero) { Console.WriteLine("Failed to find window!"); } else { Console.WriteLine($"Window handle found: {hWnd}"); } }}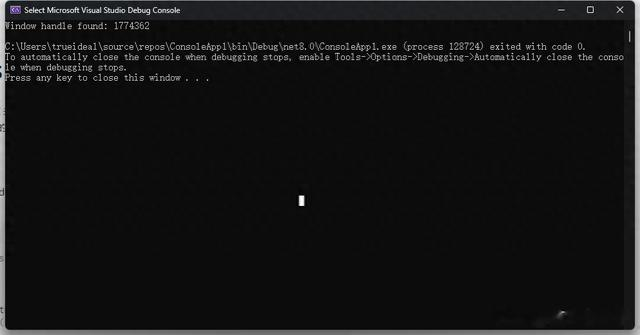
在上面的示例中,我们使用了DllImport特性来声明FindWindow函数的签名,然后在Main方法中调用该函数来查找指定类名和窗口名的窗口,并返回其句柄。
获取当前活动窗口句柄我们可以使用Windows API中的GetForegroundWindow函数来获取当前活动窗口的句柄。下面是一个简单的示例代码:
GetForegroundWindow是一个用于获取当前活动窗口句柄的Windows API函数。下面是关于GetForegroundWindow函数的参数说明:
函数原型如下:
[DllImport("user32.dll", SetLastError = true)]static extern IntPtr GetForegroundWindow();GetForegroundWindow函数没有参数,它会直接返回当前活动窗口的句柄。
调用GetForegroundWindow函数会返回当前活动窗口的句柄。如果未找到当前活动窗口,则返回IntPtr.Zero。c
using System;using System.Runtime.InteropServices;class Program{ [DllImport("user32.dll", SetLastError = true)] static extern IntPtr GetForegroundWindow(); static void Main() { IntPtr hWnd = GetForegroundWindow(); if (hWnd == IntPtr.Zero) { Console.WriteLine("Failed to get foreground window handle!"); } else { Console.WriteLine($"Foreground window handle: {hWnd}"); } }}在上面的示例中,我们使用了DllImport特性来声明GetForegroundWindow函数的签名,然后在Main方法中调用该函数来获取当前活动窗口的句柄。
枚举所有窗体句柄当使用EnumWindows函数来枚举所有顶级窗口句柄时,可以按照以下示例代码进行操作:
EnumWindows是一个用于枚举所有顶级窗口的Windows API函数。下面是关于EnumWindows函数的参数说明:
函数原型如下:
[DllImport("user32.dll", SetLastError = true)]static extern bool EnumWindows(EnumWindowsProc lpEnumFunc, IntPtr lParam);lpEnumFunc:指向一个用于处理每个窗口的回调函数的指针。这个回调函数的原型是bool EnumWindowsProc(IntPtr hWnd, IntPtr lParam)。lParam:传递给回调函数的应用程序定义的参数。EnumWindows函数会遍历所有顶级窗口,并对每个窗口调用指定的回调函数。回调函数接收两个参数:窗口句柄hWnd和应用程序定义的参数lParam。
回调函数返回true表示继续枚举,返回false表示停止枚举。
using System;using System.Runtime.InteropServices;using System.Text;class Program{ [DllImport("user32.dll")] [return: MarshalAs(UnmanagedType.Bool)] private static extern bool EnumWindows(EnumWindowsProc lpEnumFunc, IntPtr lParam); [DllImport("user32.dll", SetLastError = true, CharSet = CharSet.Auto)] private static extern int GetWindowText(IntPtr hWnd, StringBuilder lpString, int nMaxCount); private delegate bool EnumWindowsProc(IntPtr hWnd, IntPtr lParam); static void Main() { EnumWindows((hWnd, lParam) => { StringBuilder windowText = new StringBuilder(256); GetWindowText(hWnd, windowText, 256); Console.WriteLine($"Window handle: {hWnd}, Title: {windowText}"); return true; // 继续枚举 }, IntPtr.Zero); }}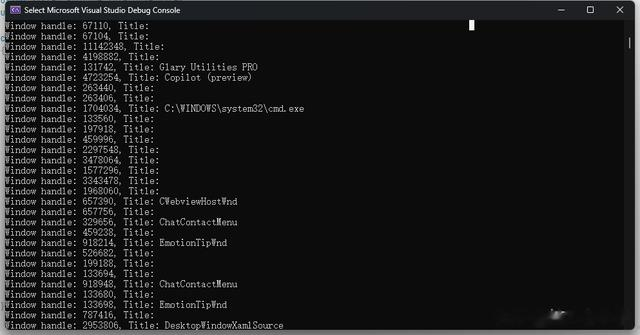
在上面的示例中,我们使用EnumWindows函数来枚举所有顶级窗口,并在回调函数中获取每个窗口的标题和句柄,然后将其输出到控制台。
通过调用EnumWindows函数,我们可以在C#中枚举所有顶级窗口的句柄,并对它们进行相应的操作。
通过调用这些Windows API函数,我们可以在C#中找到其他应用程序的窗口句柄,从而实现与其交互的操作。希望以上示例能帮助你更好地理解如何使用Windows API在C#中查找窗口句柄。