在ADO.NET中调用带输入和输出参数的存储过程,通常使用SqlCommand对象来执行存储过程,并通过其Parameters集合来设置和获取参数值。以下是一个示例,展示了如何调用一个带输入和输出参数的存储过程。
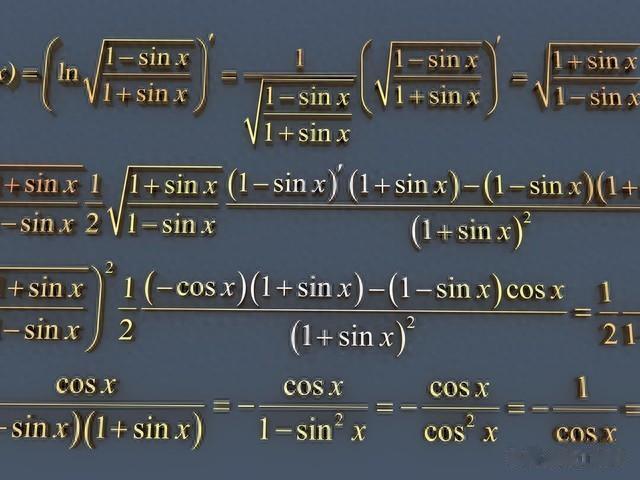
假设我们有一个存储过程GetEmployeeDetails,其定义如下:
CREATE PROCEDURE GetEmployeeDetails @EmployeeID INT, @EmployeeName NVARCHAR(100) OUTPUTASBEGIN SELECT @EmployeeName = Name FROM Employees WHERE ID = @EmployeeID;END这个存储过程接受一个输入参数@EmployeeID,并输出一个参数@EmployeeName。
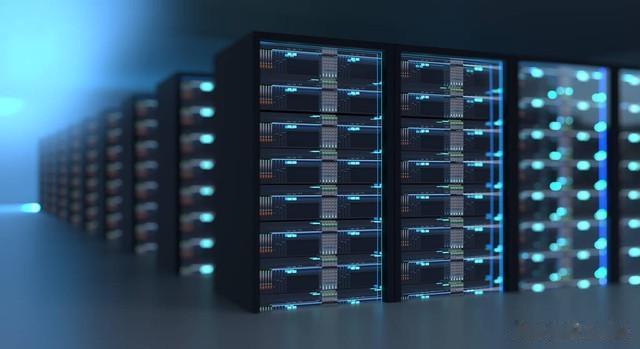
下面是使用ADO.NET调用这个存储过程的C#代码示例:
using System;using System.Data;using System.Data.SqlClient;class Program{ static void Main() { string connectionString = "your_connection_string_here"; int employeeId = 1; // 示例员工ID using (SqlConnection connection = new SqlConnection(connectionString)) { SqlCommand command = new SqlCommand("GetEmployeeDetails", connection); command.CommandType = CommandType.StoredProcedure; // 添加输入参数 SqlParameter inputParam = new SqlParameter("@EmployeeID", SqlDbType.Int); inputParam.Value = employeeId; command.Parameters.Add(inputParam); // 添加输出参数 SqlParameter outputParam = new SqlParameter("@EmployeeName", SqlDbType.NVarChar, 100) { Direction = ParameterDirection.Output }; command.Parameters.Add(outputParam); try { connection.Open(); command.ExecuteNonQuery(); // 获取输出参数的值 string employeeName = (string)outputParam.Value; Console.WriteLine($"Employee Name: {employeeName}"); } catch (Exception ex) { Console.WriteLine("An error occurred: " + ex.Message); } } }}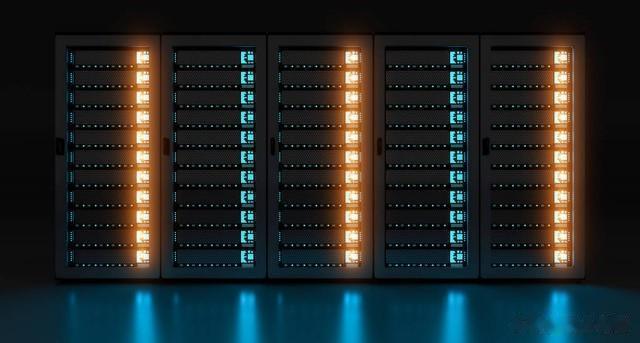
通过以上步骤,您可以使用ADO.NET成功调用带输入和输出参数的存储过程,并获取其输出值。