Python的上下文管理器是一种非常有用的特性,它允许你在代码块执行之前和之后执行特定的操作。这种机制通常用于资源管理,例如文件操作、网络连接、数据库事务等。
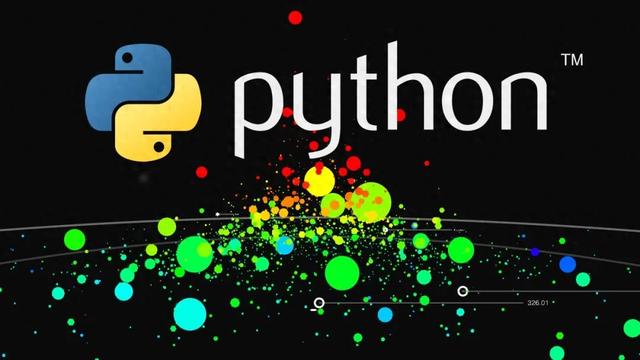
以下是一些关于上下文管理器的实用总结和技巧:
1. 上下文管理器的定义
上下文管理器是一个实现了__enter__和__exit__方法的对象。__enter__方法在进入代码块时调用,用于初始化资源。__exit__方法在退出代码块时调用,用于清理资源。class MyContextManager: def __enter__(self): print("Entering the context") def __exit__(self, exc_type, exc_value, traceback): print("Exiting the context")2. 使用with语句
使用with语句来创建一个上下文管理器,它会自动调用__enter__和__exit__方法。with语句可以确保资源在代码块执行完毕后被正确清理。with MyContextManager(): print("Inside the context")3. 内置的上下文管理器
Python标准库中提供了许多内置的上下文管理器,例如open函数用于文件操作。使用open函数作为上下文管理器可以确保文件在操作完成后被正确关闭。with open("example.txt", "r") as file: content = file.read() print(content)4. 自定义上下文管理器
可以通过实现__enter__和__exit__方法来自定义上下文管理器。在__enter__方法中可以初始化资源,在__exit__方法中可以清理资源。class MyContextManager: def __enter__(self): print("Entering the context") self.resource = "Resource" def __exit__(self, exc_type, exc_value, traceback): print("Exiting the context") del self.resource5. 上下文管理器的异常处理
在__exit__方法中,可以接收异常信息(exc_type、exc_value、traceback),并进行相应的处理。如果__exit__方法返回True,则表示异常已被处理,否则异常会继续传播。class MyContextManager: def __enter__(self): print("Entering the context") def __exit__(self, exc_type, exc_value, traceback): print("Exiting the context") if exc_type is ValueError: print("ValueError handled") return True6. 使用contextlib模块
contextlib模块提供了上下文管理器的实用工具,例如@contextmanager装饰器。使用@contextmanager装饰器可以简化上下文管理器的实现。from contextlib import contextmanager@contextmanagerdef my_context_manager(): print("Entering the context") yield print("Exiting the context")with my_context_manager(): print("Inside the context")上下文管理器是Python中一种非常有用的特性,它可以帮助你更好地管理资源,确保资源的正确初始化和清理。在实际编程中,根据需要选择合适的上下文管理器,可以提高代码的可读性和健壮性。