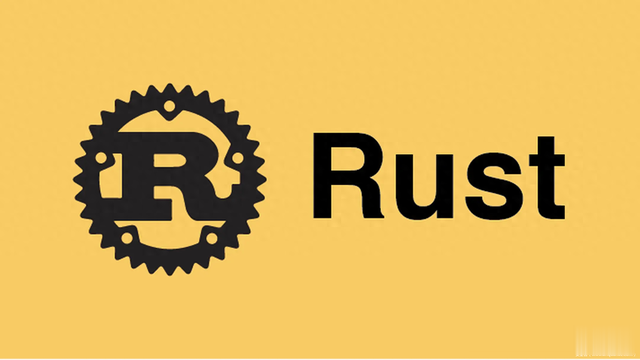
在现代异步编程场景中,处理连续数据流的需求日益普遍。无论是网络协议的分帧处理、实时数据采集,还是复杂事件流的处理,都需要一种能够有效管理异步序列的机制。Rust语言通过Stream trait提供了这一能力,其设计充分体现了Rust类型系统和所有权模型的优势。
Stream与Future的本质区别理解Stream trait的起点是将其与Future进行对比。Future表示一个异步的单一值计算,而Stream则表示一个异步的连续值序列。这种区别在它们的trait定义中表现得非常明显:
pub trait Future { type Output; fn poll(self: Pin<&mut Self>, cx: &mut Context<'_>) -> Poll<Self::Output>;}pub trait Stream { type Item; fn poll_next( self: Pin<&mut Self>, cx: &mut Context<'_> ) -> Poll<Option<Self::Item>>;}关键差异体现在Poll<Option<Item>>的返回值结构上。Stream的每次poll可能产生三种状态:
Poll::Pending:当前没有可用数据Poll::Ready(Some(item)):成功获取一个数据项Poll::Ready(None):流已终止实现自定义Stream让我们通过构建一个简单的异步计数器来实践Stream的实现。这个计数器每秒产生一个数值,直到达到设定上限:
use std::{ pin::Pin, task::{Context, Poll}, time::Duration,};use futures::Stream;use tokio::time::Sleep;struct IntervalCounter { count: u32, max: u32, delay: Sleep,}impl IntervalCounter { fn new(max: u32) -> Self { let delay = tokio::time::sleep(Duration::from_secs(1)); Self { count: 0, max, delay } }}impl Stream for IntervalCounter { type Item = u32; fn poll_next( mut self: Pin<&mut Self>, cx: &mut Context<'_> ) -> Poll<Option<Self::Item>> { if self.count >= self.max { return Poll::Ready(None); } match Pin::new(&mut self.delay).poll(cx) { Poll::Ready(_) => { self.count += 1; self.delay = tokio::time::sleep(Duration::from_secs(1)); Poll::Ready(Some(self.count)) } Poll::Pending => Poll::Pending, } }}这个实现展示了几个关键点:
内部状态管理(计数器和定时器)定时器的异步等待处理流的终止条件判断Stream的消费模式构建流只是第一步,如何有效消费流数据同样重要。常见的使用模式包括:
使用while let循环处理流
async fn process_stream() { let mut stream = IntervalCounter::new(5); while let Some(value) = stream.next().await { println!("Received value: {}", value); }}使用适配器方法
use futures::StreamExt;async fn transform_stream() { IntervalCounter::new(5) .map(|x| x * 2) .filter(|x| x % 4 == 0) .for_each(|x| async move { println!("Processed value: {}", x); }) .await;}结合select!处理多个流
use futures::{stream, StreamExt};async fn multi_stream() { let mut stream_a = IntervalCounter::new(3); let mut stream_b = stream::iter(vec!['a', 'b', 'c']); loop { tokio::select! { Some(num) = stream_a.next() => { println!("Number: {}", num); } Some(ch) = stream_b.next() => { println!("Char: {}", ch); } else => break, } }}高性能流处理策略对于需要处理高吞吐量数据流的场景,以下几个优化策略值得关注:
批量处理:使用buffered或buffer_unordered方法控制并发量背压管理:通过tokio::sync::mpsc通道实现生产者-消费者模式零拷贝处理:利用Bytes crate处理二进制数据流异步迭代器优化:使用pin-project避免不必要的内存分配以下是一个高性能流处理的示例:
use tokio_stream::StreamExt;use bytes::Bytes;async fn process_packets(stream: impl Stream<Item = Bytes>) { stream .ready_chunks(1024) // 批量处理 .for_each_concurrent(16, |batch| async move { process_batch(batch).await; }) .await;}async fn process_batch(batch: Vec<Bytes>) { // 批处理逻辑}错误处理模式健壮的流处理需要完善的错误处理机制。Rust的Result类型与Stream的结合提供了强大的错误处理能力:
use futures::TryStreamExt;async fn handle_errors(stream: impl Stream<Item = Result<u32, String>>) { stream .map_err(|e| println!("Error occurred: {}", e)) .try_for_each(|num| async move { if num % 5 == 0 { Err(format!("Invalid number {}", num)) } else { process(num).await; Ok(()) } }) .await .unwrap_or_else(|e| println!("Final error: {}", e));}实际应用场景网络协议解析
use tokio::net::TcpStream;use tokio_util::codec::{Framed, BytesCodec};async fn handle_connection(socket: TcpStream) { let mut framed = Framed::new(socket, BytesCodec::new()); while let Some(message) = framed.next().await { match message { Ok(bytes) => process_frame(bytes), Err(e) => handle_io_error(e), } }}实时数据管道
use futures::{stream, StreamExt};use tokio::sync::broadcast;async fn data_pipeline(tx: broadcast::Sender<Data>) { stream::iter(0..) .throttle(Duration::from_millis(100)) .map(|_| generate_sensor_data()) .for_each(|data| async { tx.send(data).unwrap(); }) .await;}调试与测试技巧使用tokio::time::pause控制异步时间利用StreamExt::inspect方法进行调试日志记录通过tokio_stream::StreamTest进行单元测试示例测试用例:
#[tokio::test]async fn test_stream() { let mut stream = IntervalCounter::new(3); assert_eq!(stream.next().await, Some(1)); assert_eq!(stream.next().await, Some(2)); assert_eq!(stream.next().await, Some(3)); assert_eq!(stream.next().await, None);}进阶话题展望使用async-stream crate简化流实现与tokio::sync原语结合实现复杂工作流自定义Codec实现协议编解码使用pin-project处理自引用结构与Web框架集成实现流式API响应通过深入理解Stream trait的设计哲学和实践模式,开发者可以构建出高效可靠的异步数据处理系统。Rust的类型系统保证了流处理的安全性,而丰富的生态系统提供了必要的工具支持。随着异步编程在Rust中的不断演进,Stream将继续在各种高性能场景中发挥关键作用。